[OS] Memory Layout of C Program
C program이 compile되고 난 뒤 memory에 load 될 때 layout은 다음과 같다.
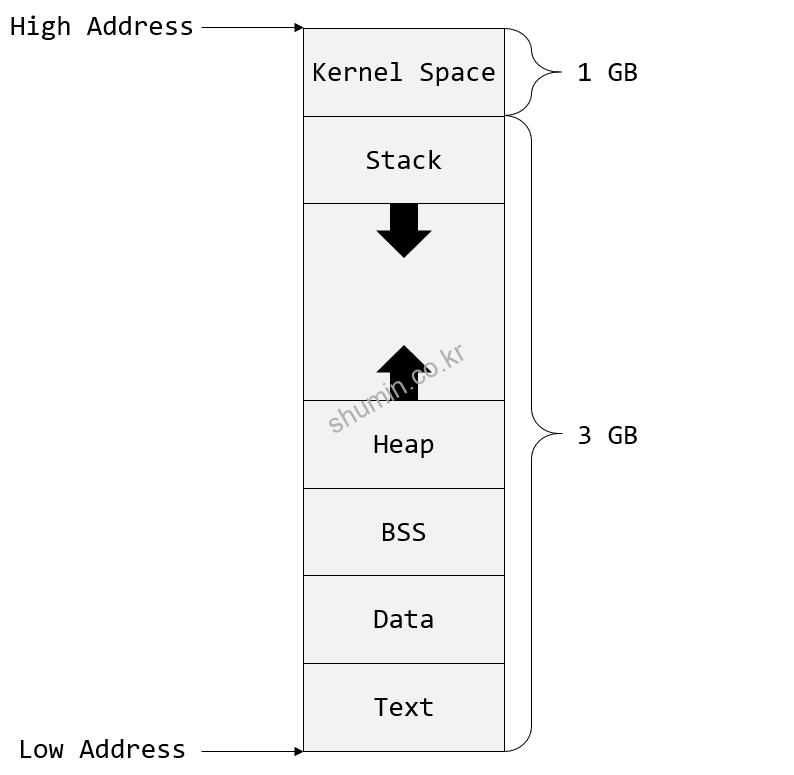
크게 총 다섯 가지 영역으로 나눌 수 있다.
- Text (Code)
- Data (Initialized)
- BSS (Uninitialized)
- Heap
- Stack
1. Text (Code) Segment
Read only 영역으로 executable instruction들이 이 영역에 위치한다.
2. Initialized Data Segment
Data segment는 초기화된 read only 영역, 그리고 초기화된 read – write 영역으로 나눠질 수 있다.
대표적인 예로 전역으로 선언된 문자열, 전역 변수는 초기화된 read – write 영역으로 할당된다. 그리고 문자열 literal인 "hello world"
와 같은 것은 read only 전용 영역에 할당된다.
#include <stdio.h> int var = 10; // Read - write data section char s1[] = "hello world" // Read - write data section void foo(void) { static int i = 10; // Read - write data section } int main(void) { foo() const char* s2 = "hello world" // Read only section }
3. Uninitialized Data Segment (BSS)
Block Started by Symbol (BSS)는 uninitialized data를 저장하는 영역으로 주로 초기화되지 않은 (또는 0) 전역변수 또는 static variable을 저장한다.
#include <stdio.h> int var = 10; // Read - write data section char s1[] = "hello world" // Read - write data section int globalVar; // BSS section void foo(void) { static int i = 10; // Read - write data section static int j; // BSS section } int main(void) { foo() const char* s2 = "hello world" // Read only section }
4. Heap Segment
Heap 영역은 필요시 동적으로 메모리를 할당하고 해지되는 영역으로, BSS section부터 address가 증가하면서 자라게 된다. 주로 malloc
, realloc
, free
등의 함수 호출로 수행된다.
그리고 모든 shared library로부터 heap section은 공유된다.
5. Stack Segment
Stack segment는 크게 다음과 같은 경우에 사용된다. Function call을 할 때는 architecure마다 차이게 있지만, stack pointer를 stack에 저장해 다시 돌아올 stack pointer 위치를 저장하는 convention을 따르면서 function call을 수행한다.
- Argument passing
- Local variable
- Return value passing
- Stack pointer
Reference
- https://blog.naver.com/PostView.nhn?blogId=cjsksk3113&logNo=222270185816
- https://www.geeksforgeeks.org/memory-layout-of-c-program/
2 Comments
ParkHyobin
BSS가 어떤 줄임말인지에 대해 오타가 있습니다. Black이 아니라 Block입니다!
shumin
답이 늦었네요, 지적 감사합니다!