[Python] 다중 상속, MRO
Python에선 다중으로 상속이 가능하다. 그래서 대표적인 상속 예시가 다이아몬드 상속이다.
class Base: def __init__(self): print('Base.__init__') class A(Base): def __init__(self): Base.__init__(self) print('A.__init__') class B(Base): def __init__(self): Base.__init__(self) print('B.__init__') class C(A,B): def __init__(self): A.__init__(self) B.__init__(self) print('C.__init__') if __name__ == '__main__': c = C()
Base.__init__ A.__init__ Base.__init__ B.__init__ C.__init__ |
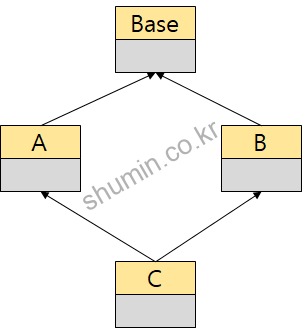
위 코드는 Base라는 최상위 class가 두 번 호출된다. C++의 경우엔 virtual이라는 것을 통해 하나라는 것을 알 수 있지만 Python은 아니다. 이를 위해서 super()
키워드가 사용된다.
class Base: def __init__(self): print('Base.__init__') class A(Base): def __init__(self): super().__init__() print('A.__init__') class B(Base): def __init__(self): super().__init__() print('B.__init__') class C(A,B): def __init__(self): super().__init__() print('C.__init__') if __name__ == '__main__': c = C()
Base.__init__ B.__init__ A.__init__ C.__init__ |
이러한 동작 원리는 Python에선 MRO (Method Resolution Order) 멤버가 존재한다.
__mro__
C.__mro__
(__main__.C, __main__.A, __main__.B, __main__.Base, object) |
실제 A와 B는 같은 level의 부모지만 각 부모 자식의 관계가 link로 연결되어있다. 즉 부모를 tracking 하는 순서가 존재한다.