[Python] Iterator
C++에선 iterator라는 STL library가 존재하며, python에선 iterator라는 객체가 존재한다.
with open("passwd") as f: try: while True: line = next(f) print(line, end='') except StopIteration: pass
hello world It’s nice |
위 예제는 특정 파일에 text를 읽어들이는 코드다. passwd 라는 파일을 읽어서 StopIteration 예외가 발생할 때까지 계속 수행한다.
items = [1, 2, 3] it = iter(items) next(it) next(it) next(it) next(it)
1 2 3 ————————————————————————————————- StopIteration Traceback (most recent call last) in —-> 1 next(it) StopIteration: |
위 코드는 iterator를 사용한 아주 기본적인 코드다. items
라는 list의 iterator 를 it
이라는 변수로 지정한다. Linked-list 구조이기 때문에 list의 next가 없을 땐 error를 발생시킨다.
Delegation 순환
Python에선 __iter__ 라는 special function이 존재한다. (__*__ 는 python에서 제공하는 special function이다.)
class Node: def __init__(self, value): self._value = value self._children = [] def __repr__(self): return 'Node({!r})'.format(self._value) def add_child(self, node): self._children.append(node) def __iter__(self): return iter(self._children) if __name__ == '__main__': root = Node(0) child1 = Node(1) child2 = Node(2) root.add_child(child1) root.add_child(child2) for ch in root: print(ch)
Node(1) Node(2) |
위 코드를 보면 __iter__
는 다음과 같이 iter()
를 호출 했을 때 수행되는 함수다.
n1 = Node()
iter(n1) # n1.__iter__()
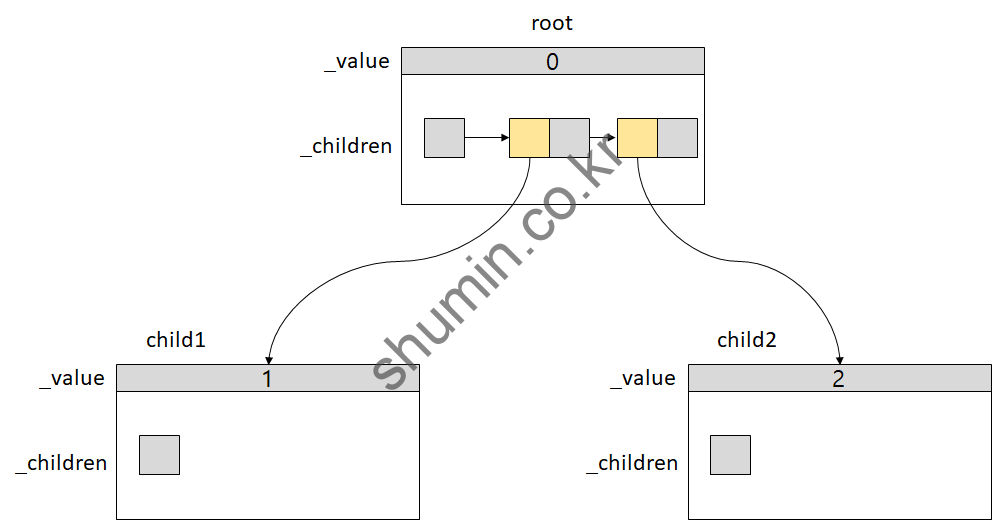
위 코드에 있는 자료 구조를 그림으로 그리면 위와 같이 될 수 있다. 코드 가장 아래에 for – in statement는 내부에 iterator를 사용하기 때문에 풀어서 보면 다음과 같이 될 수 있다.
for ch in root print(ch) ------------------- it = iter(root) while True: ch = next(it) print(ch)
print()
함수를 객체를 하게되면 원래 주소가 나오지만, __repr__
special function을 사용하게 되면 해당 함수를 호출한다.